Mastering Backend Development with Node.js: A Guide to ES6 Syntax
April 02, 2024 by clevera
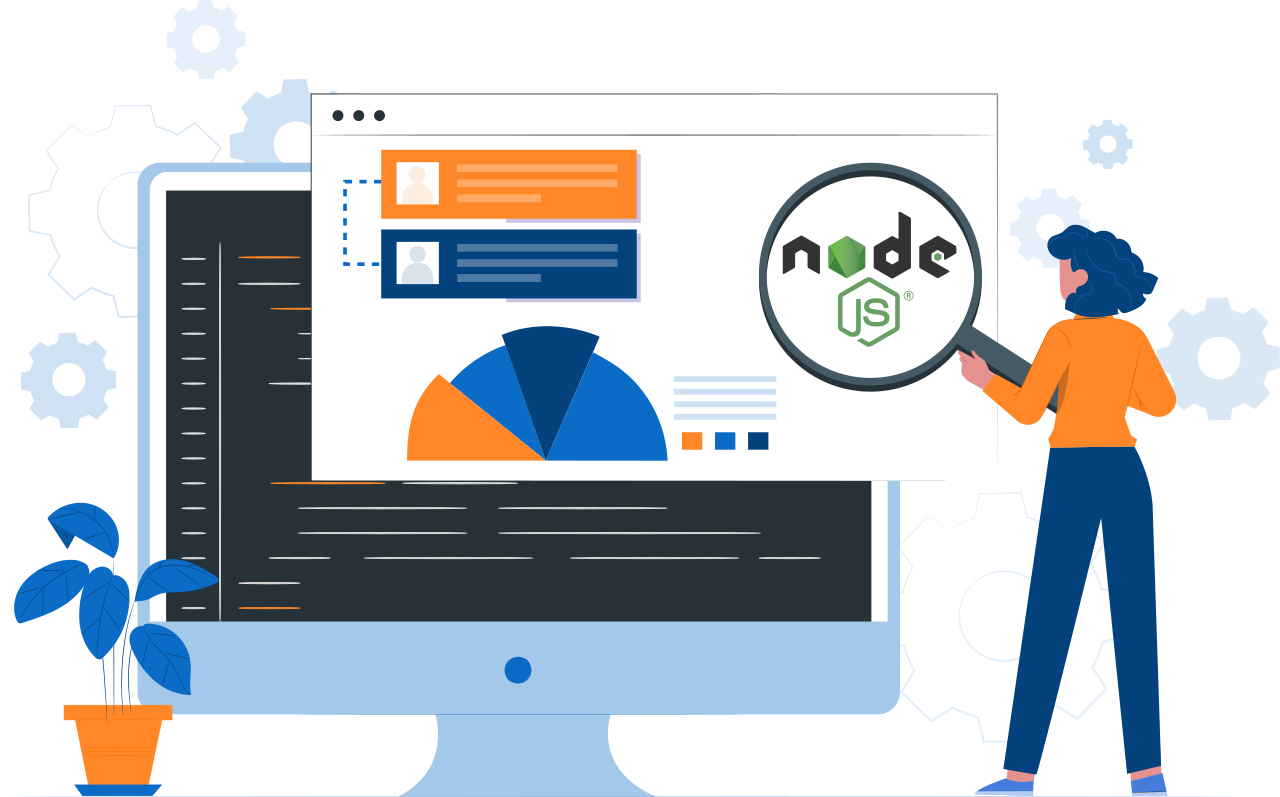
In recent years, Node.js has emerged as a powerhouse for backend development, empowering developers to build scalable and efficient server-side applications. With the advent of ES6 (ECMAScript 2015), JavaScript has undergone significant improvements, making it more expressive and powerful than ever before. In this guide, we'll explore how to leverage ES6 syntax in Node.js for seamless backend development.
Arrow Functions: ES6 introduces arrow functions, a concise way to write anonymous functions. They provide a cleaner syntax and lexical scoping, making code more readable and maintainable. In Node.js, arrow functions are widely used, especially in callbacks and event handlers.
javascript
// ES5 function
function add(a, b) {
return a + b;
}
// ES6 arrow function
const add = (a, b) => a + b;
Promises: Asynchronous programming is intrinsic to Node.js development. ES6 introduces Promises, offering a more elegant solution for managing asynchronous operations compared to callbacks. Promises simplify error handling and allow chaining multiple asynchronous operations.
javascript
// ES5 callback
function fetchData(callback) {
// Async operation
setTimeout(() => {
callback(null, data);
}, 1000);
}
// ES6 Promise
function fetchData() {
return new Promise((resolve, reject) => {
// Async operation
setTimeout(() => {
resolve(data);
}, 1000);
});
}
Destructuring: Destructuring simplifies data extraction from arrays and objects, reducing boilerplate code. In Node.js, destructuring can be particularly useful when handling request and response objects in HTTP servers.
javascript
// ES5
var user = { name: 'John', age: 30 };
var name = user.name;
var age = user.age;
// ES6
const user = { name: 'John', age: 30 };
const { name, age } = user;
Modules: ES6 introduces native support for modules, allowing developers to organize code into reusable components. In Node.js, modules are crucial for building scalable and maintainable applications. ES6 modules offer a cleaner syntax compared to CommonJS modules.
javascript
// ES5 CommonJS module
// math.js
module.exports = {
add: function(a, b) {
return a + b;
}
}
// ES6 module
// math.js
export const add = (a, b) => a + b;
// app.js
import { add } from './math.js';
Classes: ES6 brings class syntax to JavaScript, enabling developers to write object-oriented code more intuitively. In Node.js, classes can be used to create custom data structures, middleware, and controllers, improving code organization and readability.
javascript
// ES5 constructor function
function Person(name, age) {
this.name = name;
this.age = age;
}
Person.prototype.greet = function() {
return Hello, my name is ${this.name};
};
// ES6 class
class Person {
constructor(name, age) {
this.name = name;
this.age = age;
}
greet() {
return Hello, my name is ${this.name};
}
}
By embracing ES6 syntax, Node.js developers can write cleaner, more expressive code, leading to improved productivity and maintainability. Whether you're a seasoned developer or just starting with Node.js, mastering ES6 features is essential for building robust backend applications. Keep exploring and experimenting with ES6 to unlock its full potential in your Node.js projects. Happy coding!
Connect With Us
Connect
REACH US
Registered in
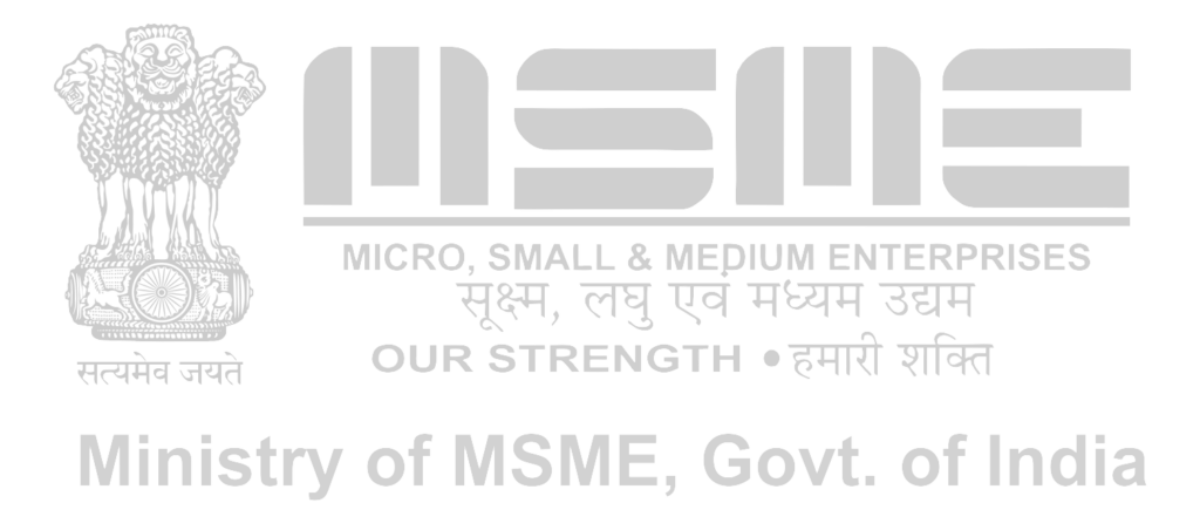
Recognized by
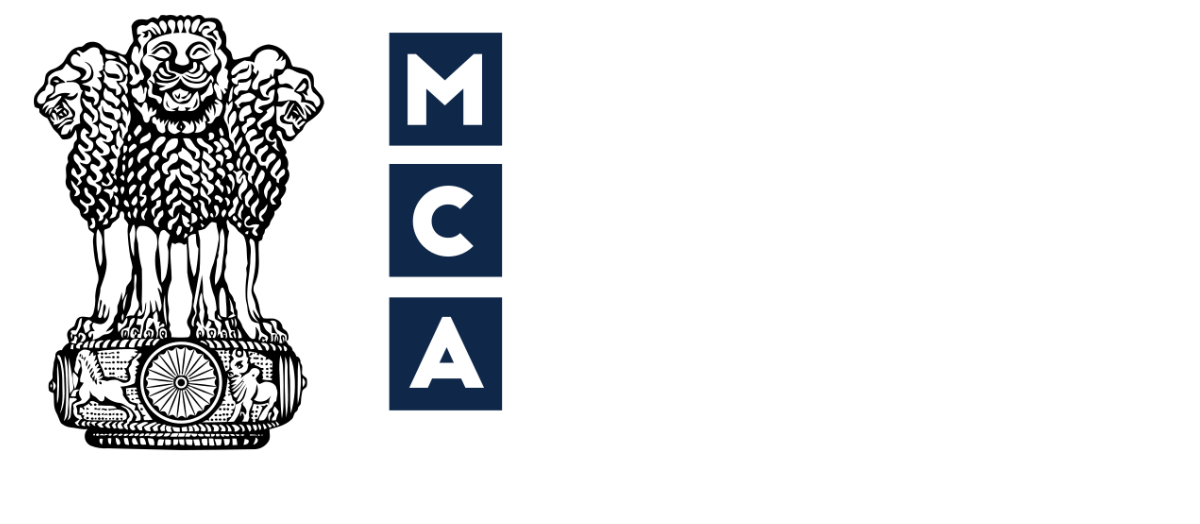
Certified by
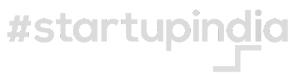
Approved by

THRIVE THROUGH CLEVERNESS